FE/Vue.js
[Vue.js 3] vuex 예제
멍목
2023. 10. 29. 14:24
반응형
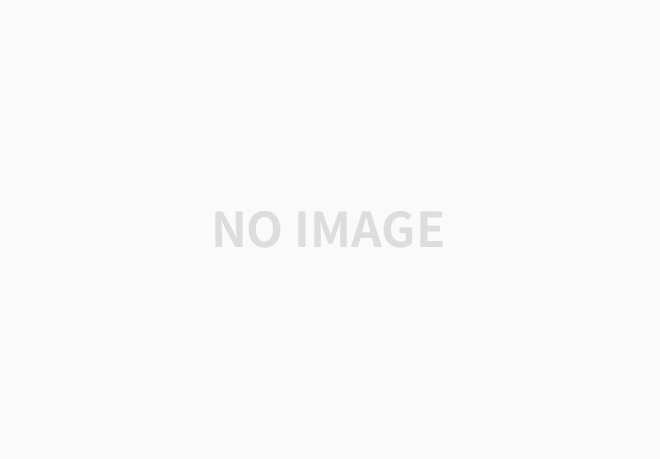
이 포스팅에서 작성하는 내용은 프로젝트로 배우는 Vue.js 3 에서 발췌하였습니다.
https://inf.run/sxQT
프로젝트로 배우는 Vue.js 3 - 인프런 | 강의
Vue.js 3 사용 방법을 배우고 프로젝트에 적용을 하면서 익힐 수 있도록 도와드립니다., 최신 Vue 3로 만나는,쉽고 강력한 프론트엔드 개발 입문! 강의 소개 [사진] 인기 프론트엔드 프레임워크, Vue.j
www.inflearn.com
vuex?
- state 관리 툴
- state를 vuex라는 저장소에서 관리하여 다른 컴포넌트에서 간편하게 접근 가능
- 즉, state를 다른 컴포넌트로 보내주거나 받을 필요 없이 단순하게 vuex 저장소에 들어가서 접근할 수 있는 툴
Vuex가 무엇인가요? | Vuex
Vuex가 무엇인가요? Vuex는 Vue.js 애플리케이션에 대한 상태 관리 패턴 + 라이브러리 입니다. 애플리케이션의 모든 컴포넌트에 대한 중앙 집중식 저장소 역할을 하며 예측 가능한 방식으로 상태를
v3.vuex.vuejs.org
vuex 설치 및 설정
npm or yarn 설치
npm install vuex --save
yarn add vuex
vuex 설정
- vue 디렉토리에
store
라는 디렉토리를 생성 후 그 안에 index.js 파일 생성 index.js
파일에 아래와 같이 입력-
import { createStore } from 'vuex'; export default createStore({ state: { } });
- vue 디렉토리의
main.js
에 아래와 같이 store를 import, use -
... import store from './store'; createApp(App).use(store).use(router).mount('#app'); // .use(store) 추가
vuex 사용
- store/index.js
파일의 state에 사용할 변수 및 mutations, actions, getters 추가
import { createStore } from 'vuex';
export default createStore({
state: {
state1: '',
state2: false
},
mutations: { // UPDATE 함수 정의
UPDATE_STATE1 (state, payload) {
state.state1 = payload;
},
UPDATE_STATE2 (state, payload) {
state.state2 = payload;
}
},
actions: { // UPDATE 함수를 조합해서 custom 함수 정의
updateAllState({ commit }, state1, state2) {
// mutations을 호출 시, commit 이용
commit('UPDATE_STATE1', state1);
commit('UPDATE_STATE2', state2);
},
getters: { // state를 가져오는 custome 함수 정ㅈ의
getState1StartByHello (state) {
return 'Hello!' + state.state1;
}
}
});
- 사용할 컴포넌트에서 useStore
import 및 예제
<script>
...
import { computed } from 'vue';
import { useStore } from 'vuex';
export default {
...
setup() {
...
const store = useStore();
// 값을 가져오기만 하고, 값이 변경된 경우에도 기존 값을 그대로 가짐
const state1 = store.state.state1;
const state2 = store.state.state2;
console.log('----- 변경 전 -----');
console.log(store.state.state1); // ''
console.log(store.state.state2); // false
// 변경을 감지해서 변경된 값을 가져오게 설정
const computedState1 = computed(() => store.state.state1);
const computedState2 = computed(() => store.state.state2);
console.log(computedState1); // ''
console.log(computedState2); // false
// actions 함수를 호출 시, dispatch 이용 (store/index.js 정의)
store.dispatch('updateAllState', 'test', true);
console.log('----- 변경 후 -----');
console.log(state1); // ''
console.log(state2); // false
console.log(computedState1); // 'test'
console.log(computedState2); // true
console.log('getters - getState1StartByHello');
const hello = store.getters.getState1StartByHello;
console.log(hello); // Hello!test
...
}
}
- store/index.js
파일에 그룹별로 설정해보기 - modules
store/index.js
import { createStore } from 'vuex';
export default createStore({
modules: {
group1: {
namespaced: true, // namespaced 를 true로 설정해야 group1에 접근 가능
state: {
state1: '',
state2: false
},
mutations: { // UPDATE 함수 정의
UPDATE_STATE1 (state, payload) {
state.state1 = payload;
},
UPDATE_STATE2 (state, payload) {
state.state2 = payload;
}
},
actions: { // UPDATE 함수를 조합해서 custom 함수 정의
updateAllState({ commit }, state1, state2) {
// mutations을 호출 시, commit 이용
commit('UPDATE_STATE1', state1);
commit('UPDATE_STATE2', state2);
},
getters: { // state를 가져오는 custome 함수 정ㅈ의
getState1StartByHello (state) {
return 'Hello!' + state.state1;
}
}
}
}
});
- 사용할 컴포넌트
<script>
...
import { computed } from 'vue';
import { useStore } from 'vuex';
export default {
...
setup() {
...
const store = useStore();
// 변경을 감지해서 변경된 값을 가져오게 설정
const computedState1 = computed(() => store.state.group1.state1);
const computedState2 = computed(() => store.state.group1.state2);
console.log(computedState1); // ''
console.log(computedState2); // false
// actions 함수를 호출 시, dispatch 이용 (store/index.js 정의)
store.dispatch('group1/updateAllState', 'test', true);
console.log('----- 변경 후 -----');
console.log(computedState1); // 'test'
console.log(computedState2); // true
console.log('getters - getState1StartByHello');
const hello = store.getters.['group1/getState1StartByHello'];
console.log(hello); // Hello!test
...
}
}
반응형