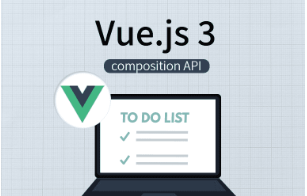
이 포스팅에서 작성하는 내용은 프로젝트로 배우는 Vue.js 3 에서 발췌하였습니다.
프로젝트로 배우는 Vue.js 3 - 인프런 | 강의
Vue.js 3 사용 방법을 배우고 프로젝트에 적용을 하면서 익힐 수 있도록 도와드립니다., - 강의 소개 | 인프런
www.inflearn.com
Vue 3 프로젝트 설치 방법
1. node.js (LTS 버전) 설치
npm 설치를 위함이며, 설치가 되어있는 경우 패스
2. cmd(Windows OS) / termial(MacOS) 에 아래의 명령어 입력
npm install -g @vue/cli
3. vue cli 버전 확인
참고) 버전 4.5 이상만 vue 3 설치 가능
vue -V
4. 아래의 명령어로 vue3 프로젝트 설치
설치 중간에 Vue 버전 선택하는 부분에서 3 선택
vue create hello-vue3
vue 파일 구조
- <template></template> : html 코드 영역
- <script></script> : javascript, typescript 코드로 이루어진 로직 영역
- <style></style> : css 코드 영역
Composition API
- Composition API : setup() Function 에서 로직을 구현하는 구조
Options API : data(), computed, watch, methods 로 이루어진 구조 - Vue3 에서도 Options API 방식을 그대로 사용할 수 있지만, 이번 프로젝트에서는 새로운 방식인 CompositionAPI 방식을 사용
- setup() 안에 변수, 함수를 만들고, 그 변수, 함수를 return 하면 해당 변수와 함수는 template 영역에서 접근 가능
template 영역에서 접근 하는 경우, {{ }} 사용
<template>
<div>{{ name }}</div>
<div>{{ helloStr('Spider Man') }}</div>
</template>
<script>
export default {
setup() {
const name = 'Iron Man';
const helloStr = (str) => {
return 'hello! ' + str;
};
return {
name,
helloStr
};
}
}
</script>
<style>
...
</style>
Fragment
<template> 영역에서 하나의 div로 감싸줘야 했는데, vue 3에서는 해주지 않아도 된다.
<template>
<div>hello</div>
<div>vue3</div>
<template>
ref, reactive
- Composition API 에서는 변수가 기본적으로 반응형 X
반응형 : 변수의 값이 변하면 template의 html에도 변경사항이 적용되는 것 - 반응형 변수로 만들어주기 위해서는 ref / reactive 사용
- ref : 문자열, 상수, 객체, 배열 모두 가능하며, value로 값에 접근
- reacitve : 객체, 배열이 가능하며, 바로 값에 접근
<template>
<div>{{ name1 }}</div>
<div>{{ name2 }}</div>
<button
class="btn btn-primary"
v-on:click="updateName"
>
이름 변경
</button>
</template>
<script>
import { ref, reactive } from 'vue';
export default {
setup() {
let name1 = ref('Iron Man 1'); // ref
let name2 = reactive({'name': 'Iron Man 2'}); // reactive
const updateName = () => {
name1.value = 'Dr.Strange 1'; // ref update
name2.name = 'Dr.Strange 2'; // reactive update
};
return {
name1,
name2,
updateName
};
}
}
</script>
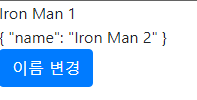
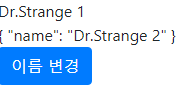
'FE > Vue.js' 카테고리의 다른 글
[Vue.js 3] Router / Event Bubbling / 객체 비교 (0) | 2023.07.30 |
---|---|
[Vue.js 3] Computed, async / await, watchEffect, watch (0) | 2023.07.16 |
[Vue.js 3] v-bind / v-model, v-for, v-show / v-if, emit / props (0) | 2023.07.09 |
[Vue.js] Vue.js 기본 문법 (0) | 2023.05.22 |
[Vue.js] vue.js 프로젝트 설정 (0) | 2023.05.21 |
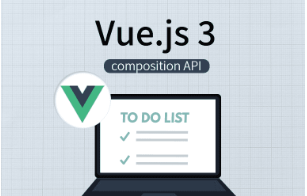
이 포스팅에서 작성하는 내용은 프로젝트로 배우는 Vue.js 3 에서 발췌하였습니다.
프로젝트로 배우는 Vue.js 3 - 인프런 | 강의
Vue.js 3 사용 방법을 배우고 프로젝트에 적용을 하면서 익힐 수 있도록 도와드립니다., - 강의 소개 | 인프런
www.inflearn.com
Vue 3 프로젝트 설치 방법
1. node.js (LTS 버전) 설치
npm 설치를 위함이며, 설치가 되어있는 경우 패스
2. cmd(Windows OS) / termial(MacOS) 에 아래의 명령어 입력
npm install -g @vue/cli
3. vue cli 버전 확인
참고) 버전 4.5 이상만 vue 3 설치 가능
vue -V
4. 아래의 명령어로 vue3 프로젝트 설치
설치 중간에 Vue 버전 선택하는 부분에서 3 선택
vue create hello-vue3
vue 파일 구조
- <template></template> : html 코드 영역
- <script></script> : javascript, typescript 코드로 이루어진 로직 영역
- <style></style> : css 코드 영역
Composition API
- Composition API : setup() Function 에서 로직을 구현하는 구조
Options API : data(), computed, watch, methods 로 이루어진 구조 - Vue3 에서도 Options API 방식을 그대로 사용할 수 있지만, 이번 프로젝트에서는 새로운 방식인 CompositionAPI 방식을 사용
- setup() 안에 변수, 함수를 만들고, 그 변수, 함수를 return 하면 해당 변수와 함수는 template 영역에서 접근 가능
template 영역에서 접근 하는 경우, {{ }} 사용
<template>
<div>{{ name }}</div>
<div>{{ helloStr('Spider Man') }}</div>
</template>
<script>
export default {
setup() {
const name = 'Iron Man';
const helloStr = (str) => {
return 'hello! ' + str;
};
return {
name,
helloStr
};
}
}
</script>
<style>
...
</style>
Fragment
<template> 영역에서 하나의 div로 감싸줘야 했는데, vue 3에서는 해주지 않아도 된다.
<template>
<div>hello</div>
<div>vue3</div>
<template>
ref, reactive
- Composition API 에서는 변수가 기본적으로 반응형 X
반응형 : 변수의 값이 변하면 template의 html에도 변경사항이 적용되는 것 - 반응형 변수로 만들어주기 위해서는 ref / reactive 사용
- ref : 문자열, 상수, 객체, 배열 모두 가능하며, value로 값에 접근
- reacitve : 객체, 배열이 가능하며, 바로 값에 접근
<template>
<div>{{ name1 }}</div>
<div>{{ name2 }}</div>
<button
class="btn btn-primary"
v-on:click="updateName"
>
이름 변경
</button>
</template>
<script>
import { ref, reactive } from 'vue';
export default {
setup() {
let name1 = ref('Iron Man 1'); // ref
let name2 = reactive({'name': 'Iron Man 2'}); // reactive
const updateName = () => {
name1.value = 'Dr.Strange 1'; // ref update
name2.name = 'Dr.Strange 2'; // reactive update
};
return {
name1,
name2,
updateName
};
}
}
</script>
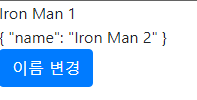
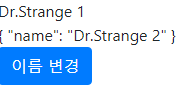
'FE > Vue.js' 카테고리의 다른 글
[Vue.js 3] Router / Event Bubbling / 객체 비교 (0) | 2023.07.30 |
---|---|
[Vue.js 3] Computed, async / await, watchEffect, watch (0) | 2023.07.16 |
[Vue.js 3] v-bind / v-model, v-for, v-show / v-if, emit / props (0) | 2023.07.09 |
[Vue.js] Vue.js 기본 문법 (0) | 2023.05.22 |
[Vue.js] vue.js 프로젝트 설정 (0) | 2023.05.21 |